Dealing with PHP encoding oddities when deserialising JSON
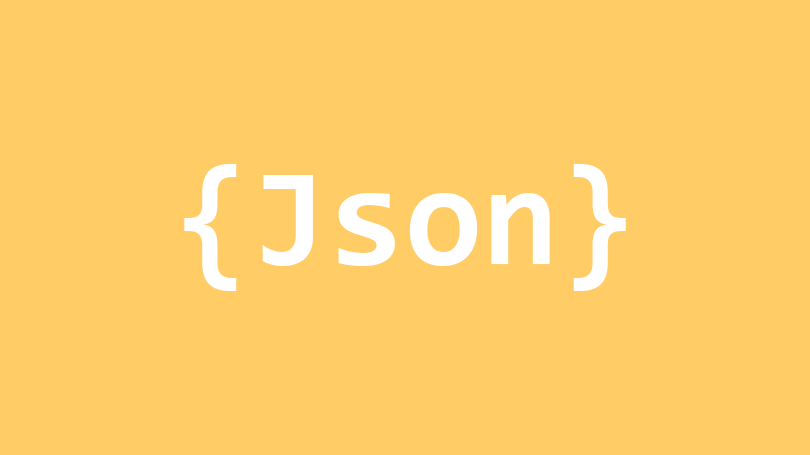
I’ve recently been consuming APIs written in PHP and found an unexpected behaviour in the JSON returned.
It appears the default behaviour for PHP is to automatically convert an empty object ("bacon" : {}
) into an empty array ("bacon" : []
).
Apart from being quite confusing this adds an extra complication when deserialising to strongly typed objects. When the object returned is populated, deserialising works fine. However trying to deserialise an empty array will throw an exception.
Custom JsonConverter in Json.Net
Json.Net allows you to create custom converters to deal with non standard behaviour such as this. In this case I decided to use a CustomCreationConverter.
The
CustomCreationConverter<T>
is a JsonConverter that provides a way to customize how an object is created during JSON deserialization. Once the object has been created it will then have values populated onto it by the serializer.
My converter looks like this.
public class EmptyArrayToObjectConverter<T> : CustomCreationConverter<T> where T : class, new()
{
public override T Create(Type objectType)
{
return new T();
}
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, JsonSerializer serializer)
{
if (reader.TokenType == JsonToken.StartArray)
{
reader.Read();
if (reader.TokenType == JsonToken.EndArray)
return new T();
throw new JsonSerializationException($"Cannot convert non-empty JSON Array to an object type {typeof(T)}");
}
return serializer.Deserialize(reader, objectType);
}
}
In the ReadJson
method we check for an empty array and if it is return a new instance of T
. Otherwise deserialise as normal.
The JsonConverter
attribute is used on the property like so:
public class Hamburger
{
[JsonConverter(typeof(EmptyArrayToObjectConverter<Bacon>))
public Bacon Bacon { get; set; }
}
Both cases are now handled and will be deserialised to the correct type with no exceptions thrown.
Summary
Mapping Json to strongly typed objects is not always straight forward and some customisation can be needed. Luckily converters allow us to overcome any difficulties or non standard behaviour.