String to an object value converter - WinRT | Windows 8.1 store apps
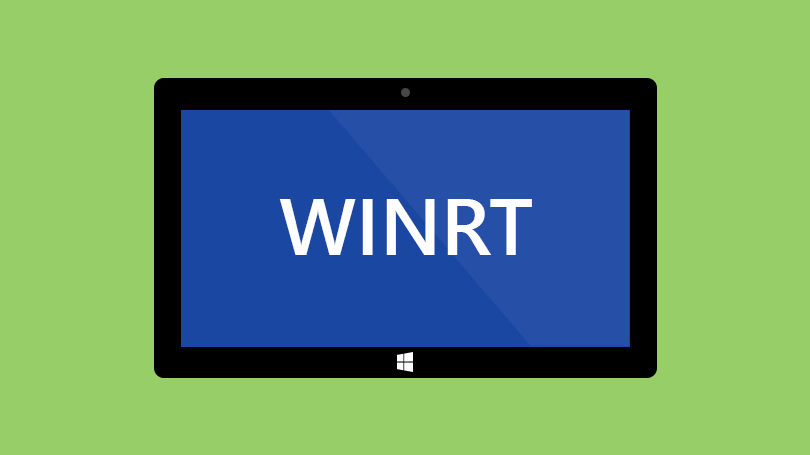
A value converter that takes a string and returns an object from a resource dictionary.
Background
I was looking for a way to show an image based on the value of a string. There were three different images I could show, one for each of the following values:
Info
Warning
Error
I could of had three different Image
controls in my XAML, each with a visibility converter, but I thought there must be a cleaner way.
The string to object converter
In my search for an alternative I found a rather neat trick. Unfortunately I can't find the source to attribute it, but who ever you are, thank you!
The trick is to add a ResourceDictionary
as a public property to our value converter class. In our class below we have added the Items
property.
[ContentProperty(Name = "Items")]
public class StringToObjectConverter : IValueConverter
{
public ResourceDictionary Items { get; set; }
public object Convert(object value, Type targetType, object parameter, string language)
{
string key = value.ToString();
return this.Items[key];
}
public object ConvertBack(object value, Type targetType, object parameter, string language)
{
throw new NotImplementedException();
}
}
The value
parameter will be a string name representing the image to be shown (Info, Warning or Error). This is the key
which we match the resources against.
The resources are set in the XAML (for our example, three images).
<valueConverters:StringToObjectConverter x:Key="Icons">
<ResourceDictionary>
<BitmapImage x:Key="Info" UriSource="ms-appx:///Assets/info.png"/>
<BitmapImage x:Key="Warning" UriSource="ms-appx:///Assets/warning.png"/>
<BitmapImage x:Key="Error" UriSource="ms-appx:///Assets/error.png"/>
</ResourceDictionary>
</valueConverters:StringToObjectConverter>
Each resource has a Key
. This is what we match against in the converter.
Finally, add the converter to the Image
.
<Image Source="{Binding ImageName, Converter={StaticResource Icons}}" />
And that's all there is to it. I can now bind a different image depending on the value of a string property on the view model.
Summary
This is a neat way to have conditional logic in your XAML. I've shown how you can use it with different images but this could be used in many different ways, colours, text, etc. Converters that have multiple uses are really handy and help keep down the number you have to write.