Windows 8.1 store apps: Creating a simple fade animation using storyboards and behaviors - WinRT
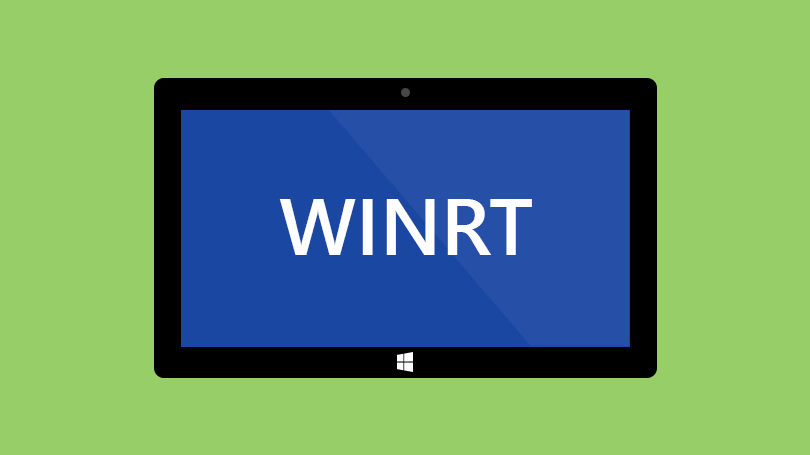
This article shows how to trigger a storyboard animation using interaction behaviors.
I needed to display a message to the user once all items in a list had been processed. Once the message had been displayed I wanted to fade this out. Here's how I achieved it.
<Border Background="Red"
x:Name="ProcessCompleteAlert"
Visibility="{Binding HasProcessComplete, Converter={StaticResource BooleanToVisibilityConverter}}">
<Border.Resources>
<Storyboard x:Name="Fading">
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(UIElement.Opacity)"
Storyboard.TargetName="ProcessCompleteAlert">
<EasingDoubleKeyFrame KeyTime="0" Value="1"/>
<EasingDoubleKeyFrame KeyTime="0:0:3" Value="1"/>
<EasingDoubleKeyFrame KeyTime="0:0:4" Value="0"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</Border.Resources>
<TextBlock Text="ALL ITEMS HAVE BEEN PROCESSED"/>
<interactivity:Interaction.Behaviors>
<core:DataTriggerBehavior Binding="{Binding HasProcessComplete}" Value="True">
<media:ControlStoryboardAction Storyboard="{StaticResource Fading}"/>
</core:DataTriggerBehavior>
</interactivity:Interaction.Behaviors>
</Border>
Firstly, to set the visibility of the message I am using a boolean to visibility converter, binding to HasProcessComplete
.
Visibility="{Binding HasProcessComplete, Converter={StaticResource BooleanToVisibilityConverter}}">
To fade the message away I created a storyboard using the DoubleAnimationsUsingKeyFrames
class and three frames to change the opacity value from 1 to 0.
<Storyboard x:Name="Fading">
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(UIElement.Opacity)"
Storyboard.TargetName="ProcessCompleteAlert">
<EasingDoubleKeyFrame KeyTime="0" Value="1"/>
<EasingDoubleKeyFrame KeyTime="0:0:3" Value="1"/>
<EasingDoubleKeyFrame KeyTime="0:0:4" Value="0"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
I wanted to run the animation when HasProcessComplete
was set to true
. Interaction behaviors allow us to do this.
<interactivity:Interaction.Behaviors>
<core:DataTriggerBehavior Binding="{Binding HasProcessComplete}" Value="True">
<media:ControlStoryboardAction Storyboard="{StaticResource Fading}"/>
</core:DataTriggerBehavior>
</interactivity:Interaction.Behaviors>
To run the storyboard I am using a DataTriggerBehaviour
binding to the HasProcessComplete
property. Now whenever the property is set to true
, the storyboard will run, fading the message out.
And there we have it, a simple and effective way to run a storyboard animation using behaviors.