How to serve static files in ASP.NET Core 2.0 MVC
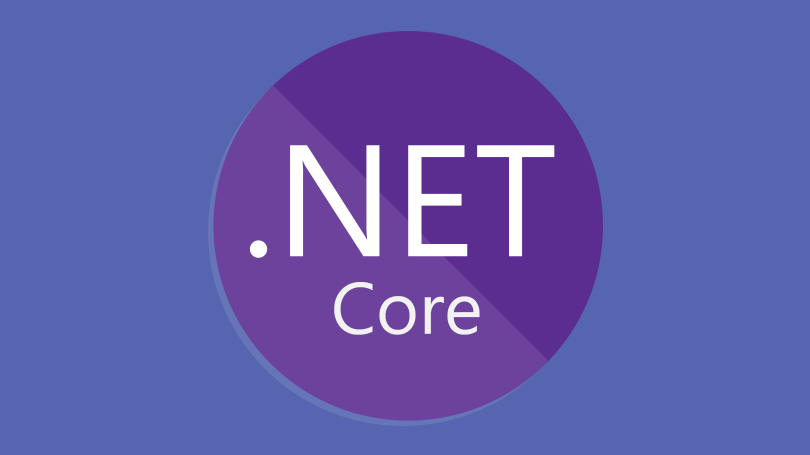
The ability to serve static files in an MVC Core app is completely optional. This article shows you how to add and configure it should you need it.
This post follows on from Create a minimal ASP.NET Core 2.0 MVC web application, worth a read if you are creating an MVC project from an empty template.
Static files
Static files can be HTML, CSS, image or JavaScript. They are located within the app and served to client (e.g. browser) directly.
Static file location
Historically the default location for static files in ASP.NET applications was the Content folder in the root of the project. However, you did not have to stick to this convention and the files could be placed anywhere really. In ASP.NET Core the default location is in the web root
(wwwroot) folder. Again, you can change this if you wish. For this article we are sticking to the default location.
Within the web root
you can have any folder structure you desire to group your files. Go crazy, as long as it makes sense to you. This is my vanilla structure.
Adding the static file handler
As I mentioned at the start static files aren't served by default, first add the Microsoft.AspNetCore.StaticFiles package to your project. Now configure the app to serve static files. Open Startup.cs
and call UseStaticFiles
from Configure
.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
// ...
}
Requesting static files
With static files configured, serving them is simply a link in the page. For example, if I wanted to serve bacon.css from the css folder the URL would be:
http://<app>/css/bacon.css
Try it out. Add a CSS file in wwwroot/css and then add a link in the homepage HTML.
<link href="css/bacon.css" rel="stylesheet">
Run the project and either browse to the URL of the style sheet or check the network tab of the browsers developer tool to check the file was served.
Summary
Serving static files is very simple, a case of adding a NuGet package and one line of code to configure the handling. Whilst initially this may seem annoying, we're used to everything being available, one of the great things about ASP.NET Core is you only add what you need. If you serve all your static content via a CDN for example, there is no need to add a static file handler. It is makes for a much leaner app.