Using Refit REST library with client-side Blazor
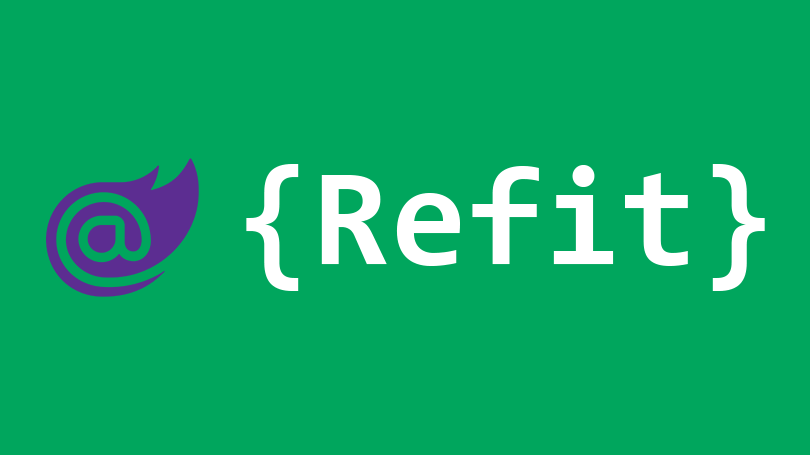
Refit, a REST library for .NET, takes your API interfaces and dynamically generates a service using HttpClient
to make the requests. This cuts out a lot of repetitive code and can be used with client-side Blazor. Here's how.
Refit
Writing code for external API requests is fairly standard and laborious. - Create a request - serialize any data to JSON - send the request - deserialize the response
Refit cuts this out. All you have to do is create an interface and let Refit do the rest. Here is an example from the Refit docs.
Create an interface.
public interface IGitHubApi
{
[Get("/users/{user}")]
Task<User> GetUser(string user);
}
Generate an implementation using the RestService
and call.
var gitHubApi = RestService.For<IGitHubApi>("https://api.github.com");
var octocat = await gitHubApi.GetUser("octocat");
Done.
Using Refit with client-side Blazor
I was happy to discover that it is possible to use Refit with client-side Blazor. The HttpClient
looks like the standard class used in .NET for HTTP requests and responses but it uses a HttpMessageHandler
called WebAssemblyHttpMessageHandler
.
All network traffic must go through the browser using the JavaScript fetch API. This handler passes the HTTP request from C# to JavaScript.
You can contine to use HttpClientFactory
and create your own typed clients as long as you use the WebAssemblyHttpMessageHandler
. To use Refit I am using Refit.HttpClientFactory
NuGet package. In Startup
I can then do this (more info in the docs)
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<WebAssemblyHttpMessageHandler>();
services.AddRefitClient<IBaconService>().ConfigureHttpClient(c =>
{
c.BaseAddress = new Uri("https://iambacon.co.uk/api");
})
.ConfigurePrimaryHttpMessageHandler<WebAssemblyHttpMessageHandler>();
}
And now Refit in Blazor works!
Summary
As I begin to use Blazor I am starting to see that it is quite flexible. This is an example of how the HttpClient
is still very configurable and that things like HttpClientFactory, Refit and Polly can still be used even though the requests and responses are going through the Fetch API in the browser.